일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
- ES6+
- 햇소
- CSS
- JS
- git
- 우아한테코톡
- github
- Python
- react
- 함수
- ES5+
- 최적화
- JavaScript
- gitCLI
- es6
- AI
- html5
- hatso
- 선택자
- object
- array
- This
- hooks
- developerlife
- next.js
- dev
- 변수
- DOM
- learn next.js
- API
- Today
- Total
codinghatso
array API (1) 본문
*API 관련 업로드는 lib.es5.d.ts 파일의 내용을 바탕으로 공부했습니다.
*파일에는 IDE(VScode)기능으로 mac은 command키 window는 control키를 누르고 원하는 함수를 클릭하면 파일이 열립니다.
toString(), toLcaleString() 문자열
/**
* Returns a string representation of an array.
*/
toString(): string;
/**
* Returns a string representation of an array. The elements are converted to string using their toLocaleString methods.
*/
toLocaleString(): string;
toString(): 해당 배열을 문자열로 return 한다.
toLcaleString(): elements(요소)가 문자열로 변환합니다.
pop(), push() 이동
/**
* Removes the last element from an array and returns it.
* If the array is empty, undefined is returned and the array is not modified.
*/
pop(): T | undefined;
/**
* Appends new elements to the end of an array, and returns the new length of the array.
* @param items New elements to add to the array.
*/
push(...items: T[]): number;
pop:
* 배열에서 마지막 요소를 제거하고 반환합니다.
* 배열이 비어 있으면 정의되지 않은 상태가 반환되고 배열이 수정되지 않습니다.
push:
* 배열 끝에 새 요소를 추가하고 배열의 새 길이를 반환합니다.
* @param 항목 어레이에 추가할 새 요소.
concat() 결합
/**
* Combines two or more arrays.
* This method returns a new array without modifying any existing arrays.
* @param items Additional arrays and/or items to add to the end of the array.
*/
concat(...items: ConcatArray<T>[]): T[];
concat:
* 둘 이상의 배열을 결합합니다.
* 이 메서드는 기존 배열을 수정하지 않고 새 배열을 반환합니다.
* @param 항목 어레이 끝에 추가할 추가 어레이 및/또는 항목.
join()
/**
* Adds all the elements of an array into a string, separated by the specified separator string.
* @param separator A string used to separate one element of the array from the next in the resulting string. If omitted, the array elements are separated with a comma.
*/
join(separator?: string): string;
//예제
const arr1 = ["🍎", "🍐"];
const arr2 = ["🍊", "🍋"];
const newArr = arr1.join(arr2);
console.log(newArr);
join:
* 배열의 모든 요소를 지정된 구분 문자열로 구분하여 문자열에 추가합니다.
* @param separator 배열의 한 요소와 결과 문자열의 다음 요소를 구분하는 데 사용되는 문자열입니다. 생략할 경우 배열 요소는 쉼표로 구분됩니다.
reverse() 반전이동
/**
* Reverses the elements in an array in place.
* This method mutates the array and returns a reference to the same array.
*/
reverse(): T[];
//예제
const arr1 = ["🍎", "🍐", "🍊", "🍋"];
const reverseArr = arr1.reverse();
console.log(reverseArr);
reverse:
* 배열에서 요소를 반전합니다.
* 이 메서드는 배열을 음소거하고 동일한 배열에 대한 참조를 반환합니다.
shift() 삭제
/**
* Removes the first element from an array and returns it.
* If the array is empty, undefined is returned and the array is not modified.
*/
shift(): T | undefined;
shift:
*맨 앞쪽에 있는 인덱스 포지션의 값 삭제
*비어있는 배열에는 undefined 값을 반환
unshift() 삽입
/**
* Inserts new elements at the start of an array, and returns the new length of the array.
* @param items Elements to insert at the start of the array.
*/
unshift(...items: T[]): number;
unshift:
* 배열의 시작 부분에 새 요소를 삽입하고 배열의 새 길이를 반환합니다.
* @param 항목 배열 시작 부분에 삽입할 요소.
slice() 값 지정
/**
* Returns a copy of a section of an array.
* For both start and end, a negative index can be used to indicate an offset from the end of the array.
* For example, -2 refers to the second to last element of the array.
* @param start The beginning index of the specified portion of the array.
* If start is undefined, then the slice begins at index 0.
* @param end The end index of the specified portion of the array. This is exclusive of the element at the index 'end'.
* If end is undefined, then the slice extends to the end of the array.
*/
slice(start?: number, end?: number): T[];
// 예제
const arr1 = ["🍎", "🍐", "🍊", "🍋"];
console.log(arr1.slice(0, 3));
console.log(arr1.slice(1, 4));
console.log(arr1.slice(3, 5));
slice:
* 배열의 단면 복사본을 반환합니다.
* 시작과 끝 모두에 대해 음수 인덱스를 사용하여 배열 끝으로부터의 간격띄우기를 나타낼 수 있습니다.
* 예를 들어 -2는 배열의 두 번째에서 마지막 요소를 나타냅니다.
* @param start 어레이의 지정된 부분의 시작 인덱스.
* 시작이 정의되지 않은 경우 슬라이스는 색인 0에서 시작됩니다.
* @param end 어레이의 지정된 부분에 대한 끝 인덱스입니다. 이것은 색인 '끝'에 있는 요소를 제외한다.
* 끝이 정의되지 않은 경우 슬라이스는 배열의 끝까지 확장됩니다.
-배열을 보존하고 원하는 부분만 return하여 새로운 배열을 만든다.
sort() 정렬
/**
* Sorts an array in place.
* This method mutates the array and returns a reference to the same array.
* @param compareFn Function used to determine the order of the elements. It is expected to return
* a negative value if first argument is less than second argument, zero if they're equal and a positive
* value otherwise. If omitted, the elements are sorted in ascending, ASCII character order.
* ```ts
* [11,2,22,1].sort((a, b) => a - b)
* ```
*/
sort(compareFn?: (a: T, b: T) => number): this;
sort:
* 배열을 제자리에 정렬합니다.
* 이 메서드는 배열을 음소거하고 동일한 배열에 대한 참조를 반환합니다.
* @param compareFn 함수 원소 순서를 결정하는 데 사용됩니다. 그것은 돌아올 예정이다.
* 첫 번째 인수가 두 번째 인수보다 작으면 음수 값, 같고 양수이면 0
* 다른 가치로 평가합니다. 생략할 경우 요소는 오름차순, ASCII 문자 순서로 정렬됩니다.
splice() 선택 요소 삭제, 요소 삽입
/**
* Removes elements from an array and, if necessary, inserts new elements in their place, returning the deleted elements.
* @param start The zero-based location in the array from which to start removing elements.
* @param deleteCount The number of elements to remove.
* @returns An array containing the elements that were deleted.
*/
splice(start: number, deleteCount?: number): T[];
/**
* Removes elements from an array and, if necessary, inserts new elements in their place, returning the deleted elements.
* @param start The zero-based location in the array from which to start removing elements.
* @param deleteCount The number of elements to remove.
* @param items Elements to insert into the array in place of the deleted elements.
* @returns An array containing the elements that were deleted.
*/
splice(start: number, deleteCount: number, ...items: T[]): T[];
splice:
* 배열에서 요소를 제거하고 필요한 경우 새 요소를 해당 위치에 삽입하여 삭제된 요소를 반환합니다.
* @param start 어레이에서 요소 제거를 시작할 0 기반 위치.
* @param deleteCount 제거할 요소 수.
* @param 항목 삭제된 요소 대신 배열에 삽입할 요소. [...items]
* @삭제된 요소를 포함하는 배열이 반환됩니다.
-배열 자체를 변경하는 api 배열을 보존하고 원하는 부분만 return하고 싶다면 slice()를 사용해야한다.
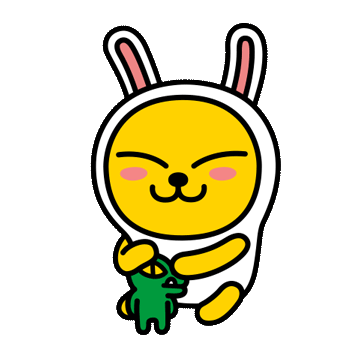
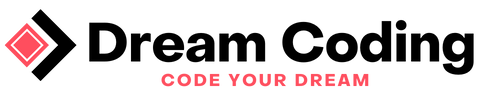
'WEB > JavaScript_Diary' 카테고리의 다른 글
JSON(JavaScript Object Notation) (0) | 2022.02.01 |
---|---|
array API (2) (0) | 2022.01.26 |
Object 개념 정리 (0) | 2022.01.25 |
node.js-Error: listen EADDRINUSE: address already in use :::5050 (0) | 2021.12.08 |
javascript 공부의 방향성에 대한 글 (0) | 2021.08.11 |