일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
- ES5+
- dev
- 최적화
- hatso
- hooks
- 변수
- This
- developerlife
- es6
- DOM
- 햇소
- JavaScript
- 우아한테코톡
- JS
- CSS
- AI
- object
- 선택자
- Python
- github
- API
- ES6+
- next.js
- learn next.js
- gitCLI
- react
- html5
- array
- 함수
- git
- Today
- Total
codinghatso
array API (2) 본문
indexOf() 검색
/**
* Returns the index of the first occurrence of a value in an array, or -1 if it is not present.
* @param searchElement The value to locate in the array.
* @param fromIndex The array index at which to begin the search. If fromIndex is omitted, the search starts at index 0.
*/
indexOf(searchElement: T, fromIndex?: number): number;
indexOf:
* 배열에서 값이 처음 나타나는 인덱스를 반환하거나 값이 없으면 -1을 반환합니다.
* @param searchElement 어레이에서 찾을 값.
* @param fromIndex 검색을 시작할 배열 인덱스. 인덱스에서 생략하면 인덱스 0에서 검색이 시작됩니다.
lastIndexOf()
/**
* Returns the index of the last occurrence of a specified value in an array, or -1 if it is not present.
* @param searchElement The value to locate in the array.
* @param fromIndex The array index at which to begin searching backward. If fromIndex is omitted, the search starts at the last index in the array.
*/
lastIndexOf(searchElement: T, fromIndex?: number): number;s
lastIndexOf:
배열에서 지정한 값이 마지막으로 나타나는 인덱스를 반환하거나 값이 없는 경우 -1을 반환합니다.
* @param searchElement 어레이에서 찾을 값.
* @param fromIndex 거꾸로 검색을 시작할 배열 인덱스. 인덱스에서 생략하면 배열의 마지막 인덱스에서 검색이 시작됩니다.
every() 배열확인 작업
/**
* Determines whether all the members of an array satisfy the specified test.
* @param predicate A function that accepts up to three arguments. The every method calls
* the predicate function for each element in the array until the predicate returns a value
* which is coercible to the Boolean value false, or until the end of the array.
* @param thisArg An object to which the this keyword can refer in the predicate function.
* If thisArg is omitted, undefined is used as the this value.
*/
every<S extends T>(predicate: (value: T, index: number, array: T[]) => value is S, thisArg?: any): this is S[];
//예제
const numberArr = [3, 4, 5, 6];
console.log(
numberArr.every(function (x) {
return x > 4;
})
);
console.log(
numberArr.every((x) => {
return x < 7;
})
);
every:
* 배열의 모든 멤버가 지정된 테스트를 충족하는지 여부를 확인합니다.
* @param 술어 최대 3개의 인수를 허용하는 함수입니다. 모든 방법이 호출한다.
* 술어가 값을 반환할 때까지 배열의 각 요소에 대한 술어 함수
* 이는 부울 값 false 또는 배열이 끝날 때까지 강제할 수 없습니다.
* @param thisArg 이 키워드가 술어 함수에서 참조할 수 있는 개체입니다.
* 이 Arg를 생략하면 정의되지 않은 값이 이 값으로 사용됩니다.
-.every()는 배열의 모든 원소가 조건에 맞는지 검사하는 메서드입니다. 모든 원소가 조건을 만족하면 true, 하나라도 만족하지 않으면 false를 반환합니다.
some() 기능 검사 return이 ture 값이 있는지
/**
* Determines whether the specified callback function returns true for any element of an array.
* @param predicate A function that accepts up to three arguments. The some method calls
* the predicate function for each element in the array until the predicate returns a value
* which is coercible to the Boolean value true, or until the end of the array.
* @param thisArg An object to which the this keyword can refer in the predicate function.
* If thisArg is omitted, undefined is used as the this value.
*/
some(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): boolean;
//예제 some
const numberArr = [3, 4, 5, 6];
function someFun(number) {
return number < 2;
}
function otherFun(number) {
return number > 4;
}
console.log(numberArr.some(someFun));
console.log(numberArr.some(otherFun));
some:
* 지정한 콜백 함수가 배열의 요소에 대해 true를 반환하는지 여부를 결정합니다.
* @param 술어 최대 3개의 인수를 허용하는 함수입니다. 일부 메서드는 호출한다.
* 술어가 값을 반환할 때까지 배열의 각 요소에 대한 술어 함수
* 이는 Boolean 값 true 또는 배열의 끝까지 강제할 수 없습니다.
* @param thisArg 이 키워드가 술어 함수에서 참조할 수 있는 개체입니다.
* 이 Arg를 생략하면 정의되지 않은 값이 이 값으로 사용됩니다.
-함수의 return 조건을 만족하는지 검사 후 bool값 반환
학생 배열을 가진 사용 예제
class Student {
constructor(name, age, enrolled, score) {
this.name = name;
this.age = age;
this.enrolled = enrolled;
this.score = score;
}
}
const students = [
new Student("A", 29, true, 45),
new Student("B", 28, false, 80),
new Student("C", 30, true, 90),
new Student("D", 40, false, 66),
new Student("E", 18, true, 88),
];
find() 콜백함수 :조건에 맞는 요소 찾기
// Q5. find a student with the score 90
{
const result = students.find((student) => student.score === 90);
console.log(result);
}
/**
* Returns the value of the first element in the array where predicate is true, and undefined
* otherwise.
* @param predicate find calls predicate once for each element of the array, in ascending
* order, until it finds one where predicate returns true. If such an element is found, find
* immediately returns that element value. Otherwise, find returns undefined.
* @param thisArg If provided, it will be used as the this value for each invocation of
* predicate. If it is not provided, undefined is used instead.
*/
find<S extends T>(predicate: (this: void, value: T, index: number, obj: T[]) => value is S, thisArg?: any): S | undefined;
find(predicate: (value: T, index: number, obj: T[]) => unknown, thisArg?: any): T | undefined;
* 배열에서 술어가 참이고 정의되지 않은 첫 번째 요소의 값을 반환합니다.
* 그렇지 않으면요
* @param 서술어 찾기는 배열의 각 요소에 대해 한 번씩 오름차순으로 호출을 찾습니다.
* 순서, 술어가 true로 반환되는 순서를 찾을 때까지. 이러한 요소가 발견되면 다음을 찾으십시오.
* 해당 요소 값을 즉시 반환합니다. 그렇지 않으면 정의되지 않은 반환을 찾습니다.
* @param thisArg 제공된 경우 이 값이 의 각 호출에 대한 이 값으로 사용됩니다.
* 술어를 쓰다 제공되지 않으면 정의되지 않은 것이 대신 사용됩니다.
-콜백함수가 조건에 맞는 값을 찾아 반환. 순차적으로 배열을 탐색하며 return이 true가 되면 즉시 반환한다.
filter() 콜백함수: 조건에 맞는 (ture)인 값만 찾기
// Q6. make an array of enrolled students
{
const result = students.filter((student) => student.enrolled);
console.log(result);
}
/**
* Returns the elements of an array that meet the condition specified in a callback function.
* @param predicate A function that accepts up to three arguments. The filter method calls the predicate function one time for each element in the array.
* @param thisArg An object to which the this keyword can refer in the predicate function. If thisArg is omitted, undefined is used as the this value.
*/
filter(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): T[];
* 콜백 함수에 지정된 조건을 충족하는 배열 요소를 반환합니다.
* @param 술어 최대 3개의 인수를 허용하는 함수입니다. 필터 메서드는 배열의 각 요소에 대해 한 번씩 술어 함수를 호출합니다.
* @param thisArg 이 키워드가 술어 함수에서 참조할 수 있는 개체입니다. 이 Arg를 생략하면 정의되지 않은 값이 이 값으로 사용됩니다.
- filtering 즉 제시한 조건에 ture인 요소들을 전부 반환한다.
map() 콜백함수: 매핑하는 과정
// Q7. make an array containing only the students' scores
// result should be: [45, 80, 90, 66, 88]
{
const result = students.map((student) => student.score);
console.log(result);
}
/**
* Calls a defined callback function on each element of an array, and returns an array that contains the results.
* @param callbackfn A function that accepts up to three arguments. The map method calls the callbackfn function one time for each element in the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function. If thisArg is omitted, undefined is used as the this value.
*/
map<U>(callbackfn: (value: T, index: number, array: T[]) => U, thisArg?: any): U[];
* 배열의 각 요소에 대해 정의된 콜백 함수를 호출하고 결과를 포함하는 배열을 반환합니다.
* @param callbackfn 최대 3개의 인수를 허용하는 함수입니다. 맵 메소드는 배열의 각 요소에 대해 콜백fn 함수를 한 번씩 호출한다.
* @param thisArg 이 키워드가 콜백fn 함수에서 참조할 수 있는 개체입니다. 이 Arg를 생략하면 정의되지 않은 값이 이 값으로 사용된다.
-배열안에 들어있는 요소 한가지 한가지를 다른것으로 변환 해주는 것을 말함.
some(),every()
// Q8. check if there is a student with the score lower than 50
{
const result = students.some((student) => student.score < 50);
console.log(result);
const result2 = !students.every((student) => student.score < 50);
console.log(result2);
}
reduce() 콜백함수 or 이니셜 벨류: 누적된 값을 전달 모아놓을 때 사용
/**
* Calls the specified callback function for all the elements in an array. The return value of the callback function is the accumulated result, and is provided as an argument in the next call to the callback function.
* @param callbackfn A function that accepts up to four arguments. The reduce method calls the callbackfn function one time for each element in the array.
* @param initialValue If initialValue is specified, it is used as the initial value to start the accumulation. The first call to the callbackfn function provides this value as an argument instead of an array value.
*/
reduce(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T): T;
reduce(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T, initialValue: T): T;
// Q9. compute students' average score
{
const result = students.reduce((prev, curr) => prev + curr.score, 0);
console.log(result / students.length);
}
* 배열의 모든 요소에 대해 지정한 콜백 함수를 호출합니다. 콜백 함수의 반환 값은 누적된 결과이며, 콜백 함수에 대한 다음 호출에서 인수로 제공됩니다.
* @param callbackfn 최대 4개의 인수를 허용하는 함수. 축소 방법은 배열의 각 요소에 대해 한 번씩 콜백fn 함수를 호출한다.
* @param initialValue initialValue가 지정된 경우 누적을 시작하는 초기 값으로 사용됩니다. 콜백fn 함수에 대한 첫 번째 호출은 배열 값 대신 인수로 이 값을 제공한다.
-콜백과 이니셜 벨류를 전달하게되고, 어떤 값이 누적된 값을 전달
-reduce( (이전값 , 현재값) => 이전값 + 현재값.score, 누적 초기값);
map().filter().join() 함수형 프로그래밍 기법 (1)
// Q10. make a string containing all the scores
// result should be: '45, 80, 90, 66, 88'
{
const result = students
.map((student) => student.score)
.filter((score) => score >= 50)
.join();
console.log(result);
}
map().sort().join() 함수형 프로그래밍 기법 (2)
// Bonus! do Q10 sorted in ascending order
// result should be: '45, 66, 80, 88, 90'
{
const result = students
.map((student) => student.score)
.sort((a, b) => a - b)
.join();
console.log(result);
}
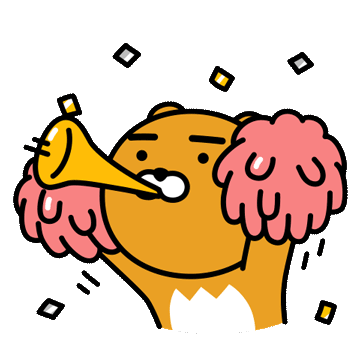

'WEB > JavaScript Diary' 카테고리의 다른 글
JS객체 정의 (0) | 2022.03.01 |
---|---|
JSON(JavaScript Object Notation) (0) | 2022.02.01 |
array API (1) (0) | 2022.01.25 |
Object 개념 정리 (0) | 2022.01.25 |
node.js-Error: listen EADDRINUSE: address already in use :::5050 (0) | 2021.12.08 |