일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- hooks
- github
- JavaScript
- dev
- Python
- ES5+
- react
- 선택자
- 변수
- html5
- 최적화
- API
- CSS
- ES6+
- next.js
- JS
- array
- 함수
- AI
- hatso
- 햇소
- learn next.js
- 우아한테코톡
- gitCLI
- DOM
- es6
- object
- git
- This
- developerlife
Archives
- Today
- Total
codinghatso
Object 개념 정리 본문
/**
* Objects
* one of the JavaScript's data types.
* a collection of related data and/or functionality.
* Nearly all objects in JavaScript are instances of Object
* object = {key : value};
*/
// 1. Literals and properties
const obj1 = {}; // 'object literal' syntax
const obj2 = new Object(); //'object constructor' syntax
function print(person) {
console.log(person.name);
console.log(person.age);
}
const hatso = { name: "hatso", age: 26 };
print(hatso);
object(객체)의 기본 형태는 key와 value 형태로 되어있습니다.
object = {key : value};
기본 적인 배열을 만드는 방법은 2가지가 있습니다.
literal(직역) 방식과 constructor(생성자) 방식
// with JavaScrpt magic (dynamically typed language)
// can add properties later
hatso.hasJob = true;
console.log(hatso.hasJob);
// can delete properties later
delete hatso.hasJob;
console.log(hatso.hasJob);
호이스팅과 관련돼있는 개념입니다. JS는 동적으로 코딩이 가능합니다.
// 2. Computed properties
// Key should be always string
console.log(hatso.name);
console.log(hatso["name"]);
hatso["hasJob"] = true;
console.log(hatso.hasJob);
function printValue(obj, key) {
console.log(obj[key]);
}
printValue(hatso, "name");
printValue(hatso, "age");
Key는 항상 문자열이어야 합니다.
printValue(hatso, "name");
printValue(hatso, "age");
이런식으로 말이죠.
// 3. Property value shorthand
const person1 = { name: "bob", age: 2 };
const person2 = { name: "steve", age: 3 };
const person3 = { name: "dave", age: 4 };
const person4 = new Person("hatso", 26);
console.log(person4);
// 4. Constructor Function
function Person(name, age) {
// this = {};
this.name = name;
this.age = age;
//return this;
}
object를 생성할 때 중복 값을 피하기 위한 방법이 있습니다.
객체 생성을 함수로 정의하여 생성하는 방법입니다. 이 방법은 처음에 언급되었던 Constructor(생성자) Function(함수) 방식입니다.
// 5. in operator: property existence check (key in obj) key가 있는지 없는지 확인 유무
console.log("name" in hatso);
console.log("age" in hatso);
console.log("random" in hatso);
console.log(hatso.random);
코드 내의 키값의 유무를 확인할 수 있습니다.
false 즉 존재 하시 않은 값을 호출 시 undefined값을 반환합니다.
// 6. for..in vs for..of
// for (key in obj)
console.clear(); // 지금까지 사용한 콘솔 출력들을 삭제한다
for (key in hatso) {
console.log(key);
}
// for (value of iterable)
const array = [1, 2, 4, 5];
for (value of array) {
console.log(value);
}
for.. in과 for.. of 기능을 하용하여 반복 호출 문을 사용할 수 있습니다.
객체의 크기가 크거나 큰 배열을 불러와야 할 때 유용할 것 같습니다.
// 7. Fun cloning
// Object.assign(dest, [obj1, obj2, obj3...])
// ref 레퍼런스가 같은 오브젝트를 가르킬때 오브젝트가 수정되면 수정된 값을 받는다.
const user = { name: "hatso", age: "20" };
const user2 = user;
user2.name = "coder";
console.log(user);
// old way
const user3 = {};
for (key in user) {
user3[key] = user[key];
}
console.clear();
console.log(user3);
//ver01.
const user4 = {};
Object.assign(user4, user);
console.log(user4);
//ver02.
const user5 = Object.assign({}, user);
console.log(user5);
// another example
const fruit1 = { color: "red" };
const fruit2 = { color: "blue", size: "big" };
const mixed = Object.assign({}, fruit1, fruit2);
console.log(mixed.color);
console.log(mixed.size);
Object의 함수를 이용하여 복제하는 방법이다.
.assign() 을 이용하면 간단하게 복제가 가능합니다.
another example에서 Object.assign을 이용해서 복제했는데 만약 중복되는 key값이 있다면 뒤쪽에 입력되는 key의 값이 덮여 쓰입니다.
*번외로 console.clear() 기능을 이용하면 호출되기 전 콘솔 출력들을 삭제합니다.
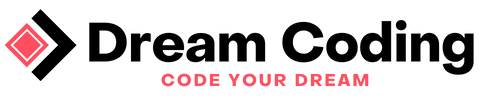
드림코딩 아카데미 → http://academy.dream-coding.com/
드림코딩 유튜브 → http://www.youtube.com/c/드림코딩by엘리
'WEB > JavaScript Diary' 카테고리의 다른 글
JSON(JavaScript Object Notation) (0) | 2022.02.01 |
---|---|
array API (2) (0) | 2022.01.26 |
array API (1) (0) | 2022.01.25 |
node.js-Error: listen EADDRINUSE: address already in use :::5050 (0) | 2021.12.08 |
javascript 공부의 방향성에 대한 글 (0) | 2021.08.11 |